由於題目有先附帳號資料,所以我們可以先把資料表建好,並直接在資料表中建立資料,進而先完成會員登入的功能;接著我們要來製作註冊會員的功能。
建立註冊畫面
front/reg.php 畫面html碼
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| <fieldset> <legend>會員註冊</legend> <span style="color:red">*請設定您要註冊的帳號及密碼(最長12個字元)</span> <table> <tr> <td class="clo">Step1:登入帳號</td> <td><input type="text" name="acc" id="acc"></td> </tr> <tr> <td class="clo">Step2:登入密碼</td> <td><input type="password" name="pw" id="pw"></td> </tr> <tr> <td class="clo">Step3:再次確認密碼</td> <td><input type="password" name="pw2" id="pw2"></td> </tr> <tr> <td class="clo">Step4:信箱(忘記密碼時使用)</td> <td><input type="text" name="email" id="email"></td> </tr> <tr> <td> <input type="button" value="註冊" onclick="reg()"> <input type="reset" value="清除" onclick="clean()"> </td> <td></td> </tr> </table> </fieldset>
|
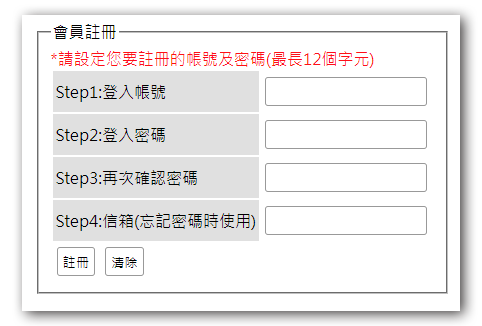
撰寫前端表單資料處理的javascript程式
清空資料函式
由於清空表單的功能在很多地方用得到,因此可以把這個函式搬到外連的 /js/js.js
中,這樣隨著頁面的引入js檔案,在任何地方都可以隨時呼叫 clean()
來使用
1 2 3 4
| function clean(){ $("input[type='text'],input[type='password'],input[type='number'],input[type='radio']").val(""); }
|
表單資料檢查及送出函式
由於題目提到要檢查帳號是否已被使用了,因此這邊使用ajax 來進行表單資料的檢查,確認申請的帳號在資料表中是不重覆,才會把表單資料送到後端去進行資料表新增會員資料的動作。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| function reg() { let user = { acc: $("#acc").val(), pw: $("#pw").val(), pw2: $("#pw2").val(), email: $("#email").val() }
if (user.acc != '' && user.pw != '' && user.pw2 != '' && user.email != '') { if (user.pw == user.pw2) { $.post("./api/chk_acc.php", {acc: user.acc}, (res) => { if (parseInt(res) == 1) { alert("帳號重覆") } else { $.post('./api/reg.php', user, (res) => { alert('註冊完成,歡迎加入') }) } }) } else { alert("密碼錯誤") } } else { alert("不可空白") } }
|
建立後端註冊程式
./api/reg.php
1 2 3 4 5 6 7 8
| include_once "db.php";
unset($_POST['pw2']);
$User->save($_POST);
|